Vue Js Get Multiple Random Element from Array:To get multiple random elements from an array in Vue.js, you can use the Math.random()
method to generate a random index within the range of the array length. Then, you can use the splice()
method to remove the element at that index and add it to a new array. Repeat this process for the desired number of random elements
How can you use Vue Js to get multiple random elements from an array?
This Vue.js method selectItems()
selects multiple random items from an array called items
and puts them into another array called selectedItems
. Here’s how it works:
- First, a copy of the original
items
array is made using the spread operator...
to avoid modifying the original array. - The
selectedItems
array is initialized as an empty array. - Then, a
for
loop is used to randomly selectnumItems
items from theremainingItems
array. - Inside the loop, a random index is generated using
Math.random()
andremainingItems.length
, which gives a random index within the range of the remaining items in the array. - The
splice()
method is then used to remove the randomly selected item from theremainingItems
array, andselectedItem
variable is assigned the value of the removed item. - Finally, the
selectedItem
is added to theselectedItems
array using thepush()
method.
Overall, this method efficiently selects multiple random items from an array without repetition, making use of array manipulation methods like splice()
and the spread operator.
Vue Js Get Multiple Random Element from Array Example
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
items: ['apple', 'banana', 'orange', 'pear', 'kiwi', 'mango'],
numItems: 3,
selectedItems: []
};
},
methods: {
selectItems() {
let remainingItems = [...this.items];
this.selectedItems = [];
for (let i = 0; i < this.numItems; i++) {
let randomIndex = Math.floor(Math.random() * remainingItems.length);
let selectedItem = remainingItems.splice(randomIndex, 1)[0];
this.selectedItems.push(selectedItem);
}
}
},
mounted() {
this.selectItems()
}
})
</script>
Output of Vue Js Get Multiple Random Element from Array
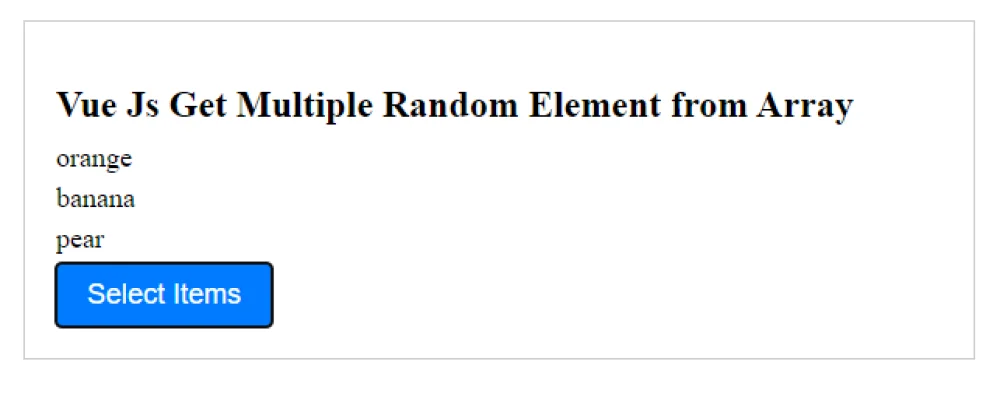